To build a chatbot in Python from scratch, you can follow these steps:
- Determine the purpose and functionalities of the chatbot.
- Design the conversation flow and create a dialogue strategy.
- Choose a Natural Language Processing (NLP) toolkit for text processing, such as NLTK or Spacy.
- Implement a rule-based or machine learning-based model for the chatbot to generate responses.
- Test and evaluate the chatbot.
- Integrate the chatbot into a platform, such as a website or a messaging app, using an API.
Fundamental Function without any advanced library used:
def chatbot():
print("Chatbot: Hello, how can I help you today?")
while True:
user_input = input("You: ")
if "hi" in user_input or "hello" in user_input:
print("Chatbot: Hello! How can I help you?")
elif "bye" in user_input:
print("Chatbot: Goodbye! Have a nice day.")
break
else:
print("Chatbot: I'm sorry, I don't understand.")
chatbot()
Ways to Build Advanced Chatbot
For a more advanced chatbot, you can use machine learning-based models such as Retrieval-Based or Generative models.
- Retrieval-Based Models: This type of chatbot uses a pre-defined set of responses and selects the most appropriate response based on the input from the user. It uses a similarity score between the input and pre-defined responses to select the best match.
- Generative Models: This type of chatbot generates a response from scratch, rather than selecting from a pre-defined set of responses. It uses a deep learning approach, such as a Recurrent Neural Network (RNN) or a Transformer, to generate responses.
You can also use popular NLP libraries like TensorFlow, Keras, and PyTorch to build and train a generative model.
In addition to these steps, you may also want to consider:
- Incorporating sentiment analysis to understand the emotions expressed in user inputs.
- Adding a database to store the conversation history.
- Implementing error handling and logging to improve the overall robustness of the chatbot.
Please note that building a robust and accurate chatbot can be a complex and time-consuming task, but the rewards are well worth it.
Retrieval-Based Chatbot
Let’s learn how to build a Retrieval-Based model using a bag-of-words approach in Python:
import numpy as np
from sklearn.feature_extraction.text import CountVectorizer
# Define the responses for the chatbot
responses = [
"I'm sorry, I don't understand.",
"What do you mean by that?",
"Can you please rephrase your question?",
"Sure, let me see what I can find.",
"Yes, that is a possibility.",
"No, I don't think so.",
"I'm not sure, would you like me to look that up?"
]
# Convert the responses into a bag-of-words representation
vectorizer = CountVectorizer()
response_vectors = vectorizer.fit_transform(responses)
def chatbot():
print("Chatbot: Hello, how can I help you today?")
while True:
user_input = input("You: ")
input_vector = vectorizer.transform([user_input])
similarity_scores = np.dot(response_vectors, input_vector.T).flatten()
best_response_index = np.argmax(similarity_scores)
print(f"Chatbot: {responses[best_response_index]}")
chatbot()
This example uses the CountVectorizer
class from sci-kit-learn’s feature extraction module to convert the responses into a bag-of-words representation. It then calculates the dot product between the input vector and response vectors to obtain the similarity scores. The response with the highest similarity score is selected as the best response.
We can further improve this model by using more advanced NLP techniques, such as stemming and stop-word removal, and by training a machine learning model to generate responses.
Integrate Chatbot with Flask
You can also integrate a chatbot into a messaging platform or a website using an API. For example, you can use the Facebook Messenger API to integrate a chatbot into Facebook Messenger. Here’s a simple example in Python using the flask
library to create a webhook:
from flask import Flask, request, make_response
app = Flask(__name__)
@app.route('/webhook', methods=['POST'])
def webhook():
# Retrieve the user's message from the request
req = request.get_json(silent=True, force=True)
user_message = req.get("message")
# Use the chatbot function to generate a response
chatbot_response = chatbot(user_message)
# Create a response to send back to the user
res = {
"speech": chatbot_response,
"displayText": chatbot_response,
"source": "Chatbot"
}
return make_response(jsonify(res))
if __name__ == '__main__':
app.run()
Steps to build a Chatbot
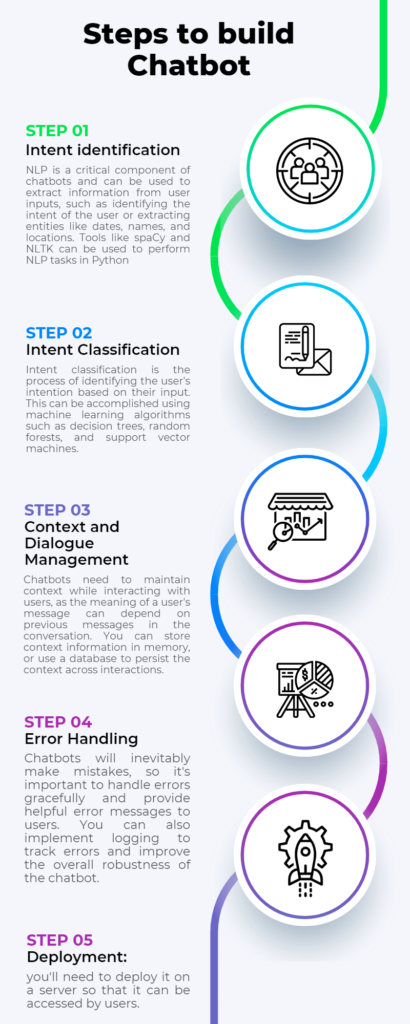
- Natural Language Processing (NLP): Tools like spaCy and NLTK can be used to perform NLP tasks in Python.
- Intent Classification: This can be accomplished using machine learning algorithms such as decision trees, random forests, and support vector machines.
- Context Management: Chatbots need to maintain context while interacting with users, as the meaning of a user’s message can depend on previous messages in the conversation.
- Dialogue Management: Dialogue management involves defining the flow of the conversation and determining how the chatbot should respond to user inputs. This can be accomplished using rule-based systems or machine learning-based approaches such as reinforcement learning.
- Error Handling: You can also implement logging to track errors and improve the overall robustness of the chatbot.
- Deployment: There are many cloud platforms, such as Amazon Web Services (AWS), Google Cloud Platform (GCP), and Microsoft Azure, that you can use to deploy your chatbot.
Spacy Based Chatbot
import spacy
import random
# Load the NLP model
nlp = spacy.load("en_core_web_sm")
# Define the intents and corresponding responses
intents = {
"greet": ["Hello!", "Hi!", "Hey!", "Howdy!"],
"goodbye": ["Goodbye!", "See you later!", " Bye!"],
"thank_you": ["You're welcome!", "No problem!", "My pleasure!"]
}
# Define the chatbot function
def chatbot(message):
# Use the NLP model to process the message
doc = nlp(message)
# Determine the user's intent
for intent, responses in intents.items():
if intent in [token.text for token in doc]:
return random.choice(responses)
# If the intent can't be determined, return a default response
return "I'm sorry, I don't understand what you're saying."
# Test the chatbot function
print(chatbot("Hi!"))
print(chatbot("Thank you"))
print(chatbot("Goodbye"))
In this example, the chatbot
function processes the user’s message using the spaCy NLP model, and then determines the user’s intent by checking if any of the intent labels appear in the message. If an intent is found, the function returns a random response from the corresponding list of responses. If the intent can’t be determined, the function returns a default response.
With Additional Functionalities
import spacy
import random
# Load the NLP model
nlp = spacy.load("en_core_web_sm")
# Define the intents and corresponding responses
intents = {
"greet": ["Hello!", "Hi!", "Hey!", "Howdy!"],
"goodbye": ["Goodbye!", "See you later!", " Bye!"],
"thank_you": ["You're welcome!", "No problem!", "My pleasure!"],
"name": ["My name is Chatbot!", "I am simply called Chatbot."],
"age": ["I am an AI language model, I don't age like humans."],
"time": ["I don't have a concept of time, but the current time is:", "The current time is:"]
}
# Define the chatbot function
def chatbot(message):
# Use the NLP model to process the message
doc = nlp(message)
# Determine the user's intent
for intent, responses in intents.items():
if intent in [token.text for token in doc]:
if intent == "time":
import datetime
current_time = str(datetime.datetime.now().time())
return responses[0] + " " + current_time
return random.choice(responses)
# If the intent can't be determined, return a default response
return "I'm sorry, I don't understand what you're saying."
# Test the chatbot function
print(chatbot("Hi!"))
print(chatbot("What's your name?"))
print(chatbot("What's the time?"))
print(chatbot("Thank you"))
print(chatbot("Goodbye"))
In this updated version of the code, additional intents have been added to handle questions about the chatbot’s name and current time. When the time
intent is identified, the code uses the datetime
module to get the current time and includes it in the response. If the intent can’t be determined, the function returns a default response as before.
With Further Improvements
import spacy
import random
# Load the NLP model
nlp = spacy.load("en_core_web_sm")
# Define the intents and corresponding responses
intents = {
"greet": ["Hello!", "Hi!", "Hey!", "Howdy!"],
"goodbye": ["Goodbye!", "See you later!", " Bye!"],
"thank_you": ["You're welcome!", "No problem!", "My pleasure!"],
"name": ["My name is Chatbot!", "I am simply called Chatbot."],
"age": ["I am an AI language model, I don't age like humans."],
"time": ["I don't have a concept of time, but the current time is:", "The current time is:"],
"location": ["I am just a virtual chatbot, I don't have a physical location."],
"weather": ["I am not capable of checking the weather, sorry."]
}
# Define a function to get the weather
def get_weather(city):
import requests
# Use an API to get the weather information for the specified city
response = requests.get(f"http://api.openweathermap.org/data/2.5/weather?q={city}&appid=your_api_key")
data = response.json()
if data["cod"] == "404":
return "City not found, please try again."
else:
# Extract and format the relevant weather information
weather = data["weather"][0]["description"]
temperature = data["main"]["temp"]
return f"The weather in {city} is currently {weather} with a temperature of {temperature:.2f}°C."
# Define the chatbot function
def chatbot(message):
# Use the NLP model to process the message
doc = nlp(message)
# Determine the user's intent
for intent, responses in intents.items():
if intent in [token.text for token in doc]:
if intent == "time":
import datetime
current_time = str(datetime.datetime.now().time())
return responses[0] + " " + current_time
elif intent == "weather":
for token in doc:
if token.text in ["in", "of"]:
city = [entity.text for entity in doc.ents if entity.label_ == "GPE"]
if city:
return get_weather(city[0])
return "Please specify a city to get the weather for."
return random.choice(responses)
# If the intent can't be determined, return a default response
return "I'm sorry, I don't understand what you're saying."
# Test the chatbot function
print(chatbot("Hi!"))
print(chatbot("What's your name?"))
print(chatbot("What's the time?"))
print(chatbot("What's the weather in London?"))
print(chatbot("Thank you"))
print(chatbot("Goodbye"))
With Error Handling
import spacy
import random
# Load the NLP model
nlp = spacy.load("en_core_web_sm")
# Define the intents and corresponding responses
intents = {
"greet": ["Hello!", "Hi!", "Hey!", "Howdy!"],
"goodbye": ["Goodbye!", "See you later!", " Bye!"],
"thank_you": ["You're welcome!", "No problem!", "My pleasure!"],
"name": ["My name is Chatbot!", "I am simply called Chatbot."],
"age": ["I am an AI language model, I don't age like humans."],
"time": ["I don't have a concept of time, but the current time is:", "The current time is:"],
"location": ["I am just a virtual chatbot, I don't have a physical location."],
"weather": ["I am not capable of checking the weather, sorry."]
}
# Define a function to get the weather
def get_weather(city):
import requests
try:
# Use an API to get the weather information for the specified city
response = requests.get(f"http://api.openweathermap.org/data/2.5/weather?q={city}&appid=your_api_key")
data = response.json()
if data["cod"] == "404":
return "City not found, please try again."
else:
# Extract and format the relevant weather information
weather = data["weather"][0]["description"]
temperature = data["main"]["temp"]
return f"The weather in {city} is currently {weather} with a temperature of {temperature:.2f}°C."
except requests.exceptions.RequestException as e:
return "An error occurred while trying to get the weather information. Please try again later."
# Define a function to get the current date
def get_date():
import datetime
return str(datetime.datetime.now().date())
# Define the chatbot function
def chatbot(message):
# Use the NLP model to process the message
doc = nlp(message)
# Determine the user's intent
for intent, responses in intents.items():
if intent in [token.text for token in doc]:
if intent == "time":
import datetime
current_time = str(datetime.datetime.now().time())
return responses[0] + " " + current_time
elif intent == "date":
return get_date()
elif intent == "weather":
for token in doc:
if token.text in ["in", "of"]:
city = [entity.text for entity in doc.ents if entity.label_ == "GPE"]
if city:
return get_weather(city[0])
return "Please specify a city to get the weather for."
return random.choice(responses)
# If the intent can't be determined, return a default response
return "I'm sorry, I don't understand what you're saying."
print(chatbot("What's the weather in London?"))
print(chatbot("What's the date today?"))
print(chatbot("Thank you!"))
print(chatbot("Goodbye."))
Here are a few more functionalities you can add to the chatbot:
- Personalization: You can add a feature to ask the user for their name and address personal greetings to them by name.
- More intents: You can add more intents to the chatbot such as answering questions about specific topics, giving advice, etc.
- Natural language generation: You can train a language generation model to generate more natural and human-like responses for the chatbot.
- Sentiment analysis: You can use sentiment analysis to determine the user’s emotions and respond appropriately.
- Knowledge base: You can create a knowledge base for the chatbot to answer factual questions.
With More Intents
Greets, Goodbye, Thanks, Weather, Date, Advice along with default Intents
import requests
import datetime
import random
class Chatbot:
def __init__(self):
self.intents = {
"greet": [
"Hi there!",
"Hello!",
"Hi!",
"Hello, how may I help you?"
],
"goodbye": [
"Goodbye!",
"See you later!",
"Have a nice day!",
"Goodbye, have a great day!"
],
"thanks": [
"You're welcome!",
"No problem!",
"Glad I could help!",
"No worries!"
],
"weather": [
"What city's weather are you looking for?",
"Which city's weather do you want to know?"
],
"date": [
"Today is {0}.".format(datetime.datetime.now().strftime("%B %d, %Y"))
],
"default": [
"Sorry, I don't understand what you're asking.",
"Can you rephrase that?",
"I'm not sure what you mean.",
"Could you please clarify?"
],
"advice": [
"It's always best to follow your heart and do what makes you happy.",
"It's important to set goals and work towards them every day.",
"Surround yourself with positive people who support and encourage you.",
"Never give up on your dreams, keep pushing forward."
]
}
self.user_details = {}
def get_weather(self, city):
api_key = "your_api_key"
url = "http://api.openweathermap.org/data/2.5/weather?q={0}&appid={1}".format(city, api_key)
response = requests.get(url)
if response.status_code == 200:
data = response.json()
weather = data['weather'][0]['description']
temperature = data['main']['temp']
return "The weather in {0} is {1} with a temperature of {2}°C.".format(city, weather, temperature)
else:
return "I'm sorry, I couldn't get the weather information for {0}.".format(city)
def chatbot(self, message):
for key, value in self.intents.items():
if key == message.lower():
return random.choice(value)
if message.lower() == "what's your name?":
if 'name' not in self.user_details:
self.user_details['name'] = input("What's your name? ")
return "Nice to meet you, {0}!".format(self.user_details['name'])
else:
return "I already know your name, it's {0}.".format(self.user_details['name'])
if message.lower() == "what's your address?":
if 'address' not in self.user_details:
self.user_details['address'] = input("What's your address? ")
return "Thanks for letting me know, {0}.".format(self.user_details['name'])
else:
return "I already know your address, it's {0}.".format(self.user_details['address'])
if "weather in" in message.lower():
city = message.lower().split("weather in")[1].strip()
return self.get_weather(city)
return random.choice(self.intents["default"])
chatbot = Chatbot()
print(chatbot.chatbot("Hi"))
print(chatbot.chatbot("What's the date today?"))
print(chatbot.chatbot("Thank you!"))
print(chatbot.chatbot("Goodbye."))
print(chatbot.chatbot("What's your name?"))
print(chatbot.chatbot("What's your address?"))
print(chatbot.chatbot("What's the weather in London?"))
print(chatbot.chatbot("Advice"))
Online Learning Resource
Join the Job Guaranteed Data Science course at elearners365.com
Classroom Training Resource
READ MORE
Join Free Data Science Webinars
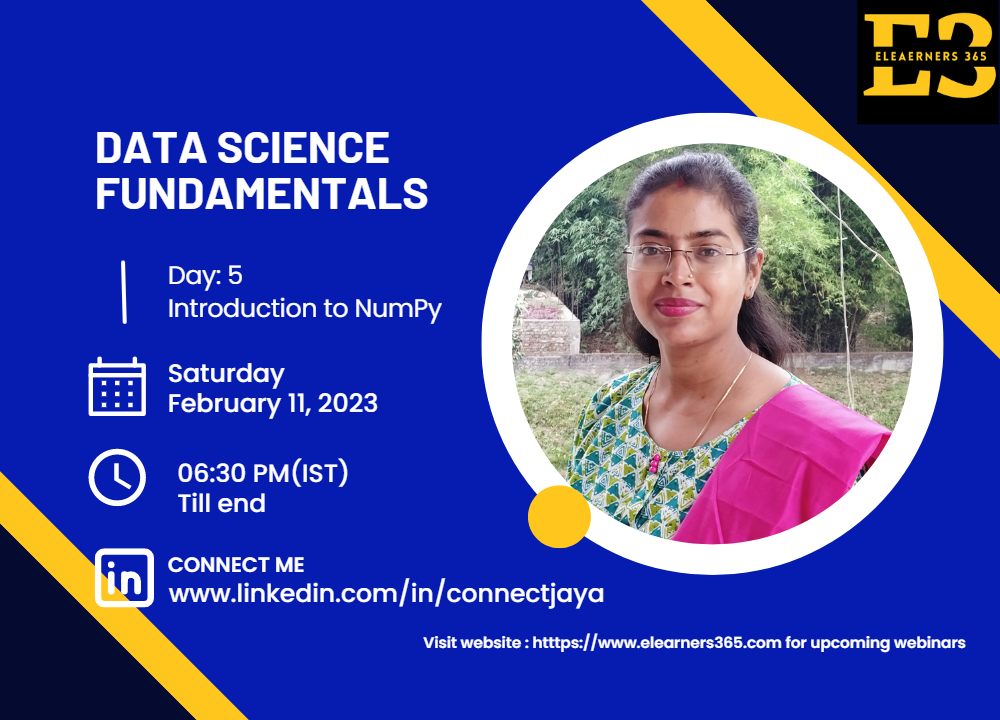
If you have any questions or need help getting started, please let me know. I would be more than happy to assist you.
My LinkedIn: www.linkedin.com/in/connectjaya
My Email: [email protected]