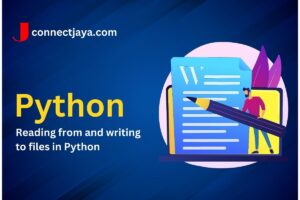
Reading from and writing to files in Python
In Python, reading from and writing to files is a common operation. This involves opening a file, performing the desired operation (reading or writing), and
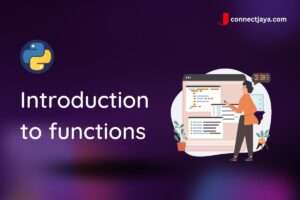
Introduction to functions
Functions are blocks of code that perform specific tasks and can be reused throughout a program. They allow you to write code that is modular,
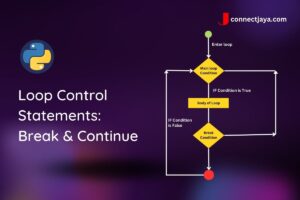
Loop Control Statements: Break & Continue
In this example, the program uses a for loop to iterate over the numbers from 1 to 10 using the range() function. Inside the loop,
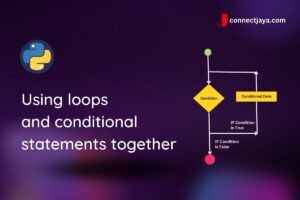
Using loops and conditional statements together
Using loops and conditional statements together in Python can be powerful and flexible. You can use a loop to iterate over a sequence of data,
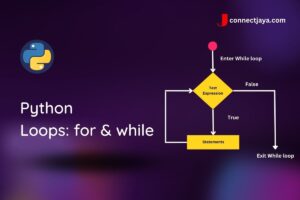
Python Loops: for & while
Loops are an important part of programming, allowing you to repeat a block of code multiple times. In Python, there are two types of loops:
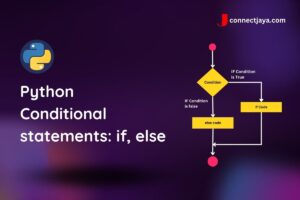
Python Conditional statements: if, else
Conditional statements allow you to execute different code blocks based on whether a condition is true or false. The basic syntax for an if/else statement
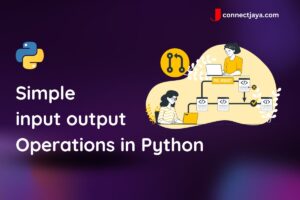
Simple input output operations in Python
1. Reading user input with input(): The input() function allows you to read user input from the console. Here’s an example: 2. Writing output to
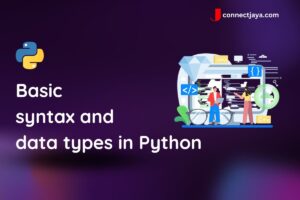
Basic syntax and data types in Python
Syntax: Python code is typically written in a text editor or integrated development environment (IDE) and saved as a file with a .py extension. The
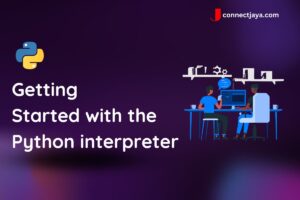
Getting started with the Python interpreter
Python provides two main ways to run Python code: the Python interpreter and Python scripts. Here’s a brief overview of each and how to use